Playmode Grid using Gizmos in Unity
Published
I was doing some work on a Mystery Dungeon-like game, with procedurally generated meshes and a grid-based movement system.
I needed to check whether my controller was using the correct cell and whether my character mesh was positioned appropriately so I tried to check using a play-mode grid.
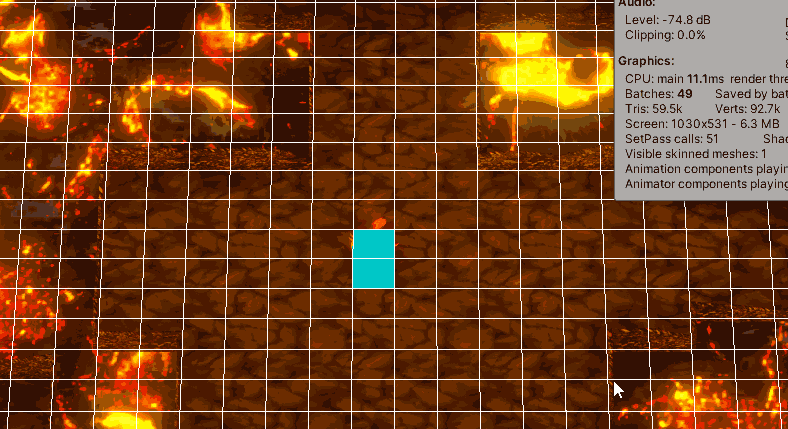
Unity doesn’t offer a grid visualizer for play mode in Editor so you have to use your own system. There are 3 main possibilities:
- Replace your ground material with a debug grid-material
- Write a shader
- Use Gizmos
In my case I decided to go with Gizmos since I was only interested with editor play-mode.
The logic is simple: create a monobehaviour and add a OnDrawGizmos function. You can also add this function to any other monobehaviour in your scene if you want to. Then, we’ll just iterate and draw lines in a grid format.
public class GridDraw: Monobehaviour {
public int minX;
public int maxX;
public int minY;
public int maxY;
void OnDrawGizmos() {
Gizmos.color = Color.white;
Vector3 pos0 = new Vector3();
Vector3 pos1 = new Vector3();
for (int i = -minX; i < maxX; i++)
{
pos0.x = i;
pos0.z = -minY;
pos1.x = i;
pos1.z = maxY;
Gizmos.DrawLine(
pos0,
pos1
);
/*for (int j = 0; j < 100; j++) {
}*/
}
for (int i = -minY; i < maxY; i++)
{
pos0.x = -minX;
pos0.z = i;
pos1.x = maxX;
pos1.z = i;
Gizmos.DrawLine(
pos0,
pos1
);
}
And that’s it for our grid! I’ll also draw a square over the current square in which my character is located. Add this code to your controller.
void OnDrawGizmos() {
Gizmos.color = Color.cyan;
Vector3 pos = new Vector3(Mathf.RoundToInt(transform.position.x), 0f, Mathf.RoundToInt(transform.position.z));
Gizmos.DrawCube(pos + new Vector3(-0.5f, 0.5f, -0.5f), new Vector3(1f, 1f, 1f));
}
The added Vector3 offsets the position to put it in the center of the cell so the square is drawn appropriately.