Implementing a Simple Camera Shake
Published
If you’re using Cinemachine, camera shake is available with it but if you aren’t you will need to implement it yourself.
Creating a basic camera shake effect is pretty simple:
- Define a duration for the shake
- Choose a strength for the effect
- Draw random coordinates between -1f and 1f, put them in a vector and normalize it
- Adjust the reference position of the camera with this normalized vector multiplied
- Repeat the random draw and the position adjustment for every frame for the specified duration
- Set the camera back to its reference position
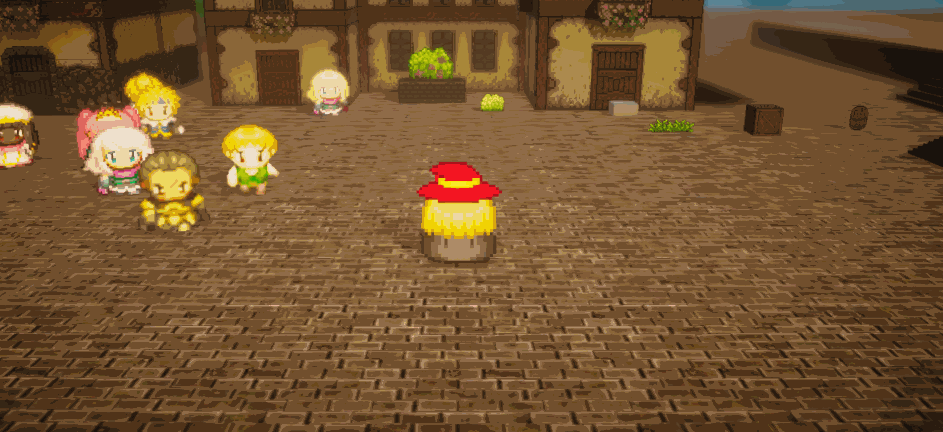
We’ll just need to put our shake logic in a coroutine. We also create a PRNG on start. The code is as follows:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CameraShaker : MonoBehaviour
{
public Vector3 mReferencePosition;
public float mShakeStrength;
public float mShakeDuration = 1f;
private System.Random _mRand;
void Start()
{
_mRand = new System.Random();
}
public void Shake() {
mReferencePosition = transform.position;
StartCoroutine(Coroutine_Shake());
}
IEnumerator Coroutine_Shake() {
float elapsed = 0f;
Vector3 displacement = new Vector3();
do {
// compute random floats between -1f and 1f
displacement.x = ((float)_mRand.NextDouble()) * 2f - 1f;
displacement.y = ((float)_mRand.NextDouble()) * 2f - 1f;
transform.position = mReferencePosition + displacement * mShakeStrength;
yield return null;
elapsed += Time.deltaTime;
} while (elapsed < mShakeDuration);
transform.position = mReferencePosition;
yield return null;
}
}
There are a lot of ways to improve this, such as adding a smoothing effect for shaking strength, I’ll leave up to you to modify this for your own purposes.
The full code is avaible in the CameraShaker.cs file on my UnitySimpleUtils repository on Github.