Querying native coins balance for tests with cw-multi-test
Published
Continuing from our previous post on creating native coins for tests, it’s also helpful to get a user’s balance to check if it has changed as we expect it in a test.
I was a bit confused since the docstring for app.raw_query made references to generated helpers for queries, but intellisense showed no relevant methods on the App type.
It’s likely I missed a trait, but instead I opted to use the raw_query and parse the resulting message myself. Queries return Binary data, which must be parsed. In the case of a balance query, the relevant type is BalanceResponse.
use cosmwasm_std::{QueryRequest, BankQuery, to_binary, from_binary, BalanceResponse};
fn query_balance_native(app: &App, address: &Addr, denom: &str) -> Coin {
let req: QueryRequest<BankQuery> = QueryRequest::Bank(BankQuery::Balance { address: address.to_string(), denom: denom.to_string() });
let res = app.raw_query(&to_binary(&req).unwrap()).unwrap().unwrap();
let balance: BalanceResponse = from_binary(&res).unwrap();
return balance.amount;
}
Update: Silly me! You actually need to call app.wrap to get access to better queries. With this in mind, hbalance query becomes a one-liner:
app.wrap().query_balance(address, denom)
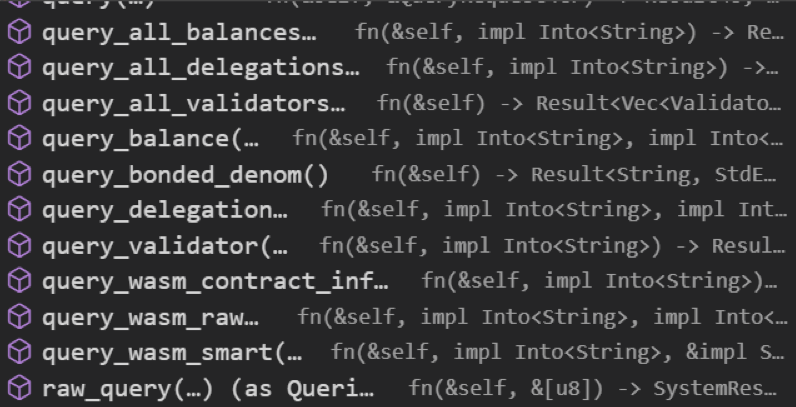